CSS Properties
CSS has quite a number of properties that will help you to make your web page appealing to look at, to add animations, and even interactivity to your website. In this module, we are going to discuss the list of CSS properties.
The properties are grouped together in alphabetical order so that it will be easier to follow along, or to look for a certain property if you're in a hurry.
Use the search bar to find specific items quickly, or use the in-built CTRL + F
browser command.
accent-color
Specifies the color to use for user-interface controls such as a checkbox, radio button or range slider.
The default accent color is light-blue, but you can change it to match the color palette of your website by doing something similar to the example below:
Example:
input[type="checkbox"] {
accent-color: #fb7185;
}
This will render a checkbox which will have a light-red color when it is checked. See the demonstration below which use checkboxes and a range slider.
align-content
This property should be used together with
display: flex
. Otherwise, it has no effect on the element in which it is defined.
Defines how flex items are distributed along the cross axis of a flexbox container.
The cross axis is the axis opposite to the default one set in the parent container. The default is usually horizonal rows, but can be changes to vertical columns.
If the container is set to rows, the cross axis becomes the column, and vice versa.
The align-content
property can take in a number of values, described below:
align-content: center
Example:
/* Parent container */
section {
display: flex;
flex-wrap: wrap;
align-content: center;
width: 100px;
height: 300px;
border: 1px solid #eeeeee;
}
/* Child elements */
section div {
width: 250px;
height: 250px;
}
This will result in the following:
align-content: flex-start
Aligns items to the start of the flex container
Example:
/* Parent container */
section {
display: flex;
flex-wrap: wrap;
align-content: flex-start;
width: 100px;
height: 300px;
border: 1px solid #eeeeee;
}
/* Child elements */
section div {
width: 250px;
height: 250px;
}
This will result in the following:
align-content: flex-end
Aligns items to the end of the flex container
Example:
/* Parent container */
section {
display: flex;
flex-wrap: wrap;
align-content: flex-end;
width: 100px;
height: 300px;
border: 1px solid #eeeeee;
}
/* Child elements */
section div {
width: 250px;
height: 250px;
}
This will result in the following:
align-content: normal
Aligns items normally in the flex container.
Example:
/* Parent container */
section {
display: flex;
flex-wrap: wrap;
align-content: normal;
width: 100px;
height: 300px;
border: 1px solid #eeeeee;
}
/* Child elements */
section div {
width: 250px;
height: 250px;
}
This will result in the following:
align-content: space-between
Places items to fit the entire width (or height depending on your cross axis) of the flex container.
Example:
/* Parent container */
section {
display: flex;
flex-wrap: wrap;
align-content: space-between;
height: 300px;
}
/* Child elements */
section div {
width: 100%;
height: 40px;
}
This will result in the following:
Notice how all the elements are evenly spaced between the parent element, starting from the top of the parent element to the bottom.
align-content: space-evenly
Places items to fit the entire width (or height depending on your cross axis) of the flex container.
Example:
/* Parent container */
section {
display: flex;
flex-wrap: wrap;
align-content: space-evenly;
height: 300px;
}
/* Child elements */
section div {
width: 100%;
height: 40px;
}
This will result in the following:
Notice how all the elements are evenly spaced evenly the parent element, but they don't start from the top. Also, the amount of space is equal all round.
align-content: space-around
Places items to fit the entire width (or height depending on your cross axis) of the flex container.
Example:
/* Parent container */
section {
display: flex;
flex-wrap: wrap;
align-content: space-around;
height: 300px;
}
/* Child elements */
section div {
width: 100%;
height: 40px;
}
This will result in the following:
Notice how all the elements are evenly spaced around the parent element, but they don't start from the top. In this case, only the space between the elements is equal. Take not of this slight difference between space-around
and space-evenly
.
align-items
This is used to define the alignment of items inside a flex or grid container. Just like align-content
, it also takes the same property values such as flex-end
, flex-start
, space-between
, space-evenly
, space-around
.
Remember to use
display: flex
ordisplay: grid
on the parent element. Otherwise,align-items
will have no effect.
Example:
/* Parent container */
section {
display: flex;
align-items: center;
}
/* Child elements */
section div {
width: 100%;
height: 40px;
}
align-self
This specifies the alignment in the block direction (downwards or top to bottom) for the selected items inside a flex a flex or grid container.
Example:
<section class="container">
<div></div>
<div class="self-div">This div has `align-self: flex-end` applied</div>
<div></div>
<div></div>
</section>
.container {
width: 100%;
height: 400px;
border: 1px solid #222222;
display: grid;
grid-template-columns: repeat(2, 1fr);
gap: 8px;
}
.container div {
width: 90%;
height: 150px;
background-color: lightblue;
}
.container .self-div {
background-color: coral;
align-self: flex-end;
}
This will result in the following:
This div has align-self: flex-end
applied
Without align-self: flex-end
applied, the result would have been the following:
Points to note
-
By default,
align-self
aligns items in the block direction (downwards). If you want to align items in the inline direction (left to right), usejustify-self
orjustify-items
instead. -
Block and inline direction depend on the language. For example, inline direction for Arabic would be right to left, and for English is left to right.
-
The
align-self
property overrides thealign-items
property. -
The
align-self
property can take in the following values:auto
,stretch
,center
,flex-start
,flex-end
,baseline
,initial
andinherit
.
all
This reselts all properties to their initial or inherited value.
Example:
html {
font-size: 14px;
color: coral;
}
p {
font-size: 20px;
color: darkgray;
}
p.reset {
all: initial;
}
This will make the paragraph that has a class of reset
to reset its current styles to the default styles of the document.
Animation properties
animation
This is used as a shorthand property to define the values of an animation.
Example:
section {
animation: spin 5s infinite;
}
The animation properties are discussed below:
animation-delay
This specifies a delay before the start of an animation.
section {
animation-delay: 2s;
}
This will start the animation after 2 seconds. The time value should be specified in seconds (s) or milliseconds (ms).
You can also use negative values for the animation delay, which will cause it to start as if it had already been playing for the amount of time specified.
Example:
section {
animation-delay: -5s;
}
animation-direction
This specifies whether the animation should be played forwards (normal), backwards(reverse) or in alternate cycles.
/* Animation goes forwards */
section {
animation-direction: normal;
}
/* Animation goes backwards */
section {
animation-direction: reverse;
}
/* Animation goes forwards and then backwards */
section {
animation-direction: alternate;
}
/* Animation goes backwards and then forwards */
section {
animation-direction: alternate-reverse;
}
animation-duration
This specifies how long the animation should take to complete one cycle.
Example:
section {
animation-duration: 5s;
}
Above, the animation will complete after 5 seconds.
animation-fill-mode
This describes the final state of your animation.
Example:
@keyframes increasefont {
from {
font-size: 16px;
}
to {
font-size: 80px;
}
}
p {
animation-name: increasefont;
animation-fill-mode: forwards;
}
This will cause the font-size
of the paragraph to increase from 16px
to 80px
and it will stay at the final font.
The default behavior for an animation is to start, end, and then revert to the
default or beginning properties. However, when you use animation-fill-mode
you can ensure that the animation will stay with the end properties.
animation-fill-mode: none
The animation will not apply any styles to the target when it's not executing. The element will instead be displayed using any other CSS rules applied to it. This is the default value.
animation-fill-mode: forwards
The target will retain the computed values set by the last keyframe encountered during execution. The last keyframe depends on the value of animation-direction
and animation-iteration-count
animation-fill-mode: backwards
The animation will apply the values defined in the first relevant keyframe as soon as it is applied to the target, and retain this during the animation-delay
period. The first relevant keyframe depends on the value of animation-direction
animation-fill-mode: both
The animation will follow the rules for both forwards and backwards, thus extending the animation properties in both directions.
animation-iteration-count
This specifies the number of times an animation should be played before stopping.
Example:
p {
animation-iteration-count: 4;
}
This will play the animation four times before stopping. You can also specify decimal values for your iteration.
The animation-iteration-count
can also take in values such as infinite
, linear
, inherit
, and initial
.
animation-play-state
This sets whether an animation is playing or is paused. When you run a paused animation, it will start from when it was paused, rather than from the beginning.
Example:
p {
animation-play-state: paused;
}
p {
animation-play-state: running;
}
animation-timing-function
This sets how an animation progresses through the duration of each cycle.
Example:
p {
animation-timing-function: linear;
}
Here is a complete reference from MDN:
/* Keyword values */
animation-timing-function: ease;
animation-timing-function: ease-in;
animation-timing-function: ease-out;
animation-timing-function: ease-in-out;
animation-timing-function: linear;
animation-timing-function: step-start;
animation-timing-function: step-end;
/* Function values */
animation-timing-function: cubic-bezier(0.1, 0.7, 1, 0.1);
animation-timing-function: steps(4, end);
/* Steps Function keywords */
animation-timing-function: steps(4, jump-start);
animation-timing-function: steps(10, jump-end);
animation-timing-function: steps(20, jump-none);
animation-timing-function: steps(5, jump-both);
animation-timing-function: steps(6, start);
animation-timing-function: steps(8, end);
/* Multiple animations */
animation-timing-function: ease, step-start, cubic-bezier(0.1, 0.7, 1, 0.1);
/* Global values */
animation-timing-function: inherit;
animation-timing-function: initial;
animation-timing-function: revert;
animation-timing-function: revert-layer;
animation-timing-function: unset;
It is always recommended to use the shorthand animation
property to define your animations, rather than having to type out all the properties you want to add in your elements every single time that you want to add an animation.
For example. rather than doing this:
p {
animation-name: play;
animation-duration: 2s;
animation-timing-function: linear;
animation-iteration-count: infinite;
}
You can do this:
p {
animation: play 2s linear infinite;
}
As you can obviously tell, it is much faster than having to type out every single property.
aspect-ratio
This defines the ratio between the width and height of an element. It is mostly used when the element often changes size and you want to preseve the ration between width and height, such as on an iframe
or a video
.
Example:
video {
aspect-ratio: 16 / 9;
}
backdrop-filter
This is used to apply a graphical effect to the area behind an element. For this property to work, the element or its background should be partially transparent.
Example:
div {
background-color: rgba(255, 255, 255, 0.4);
-webkit-backdrop-filter: blur(5px);
backdrop-filter: blur(5px);
}
-webkit-backdrop-filter
is an example of a Vendor Prefix. Read more about
Vendor Prefixes in the module, CSS Vendor Prefixes
backface-visibility
This defines whether the back of an element should be visible to the user or not. This is especaially useful when you want to rotate an element, for example when you want a profile card to rotate to reveal more information when a user hovers over it with the mouse.
Example:
div {
-webkit-backface-visibility: visible;
backface-visibility: visible;
}
-webkit-backface-visibility
is an example of a Vendor Prefix. Read more
about Vendor Prefixes in the module, CSS Vendor Prefixes
Background properties
background
This is used to set different background properties. It is a shorthand property for:
background-color
background-image
background-position
background-size
background-repeat
background-origin
background-clip
background-attachment
Example:
div {
background: red;
width: 100%;
height: 40px;
}
This will give the div
a red background color.
background-attachment
This defines whether a background image will scroll with the rest of the webpage or not.
Example:
body {
background-image: url("https://fastly.picsum.photos/id/42/200/300.jpg?hmac=RFAv_ervDAXQ4uM8dhocFa6_hkOkoBLeRR35gF8OHgs);
background-repeat: no-repeat;
background-attachment: fixed;
}
The image above will scroll with the rest of the webpage.
background-blend-mode
This specifies the how background layers, such as colors or images, should be blended with one another.
Example:
div {
background-repeat: no-repeat, repeat;
background-image: url("img_tree.gif"), url("paper.gif");
background-blend-mode: lighten;
}
Other values that can be used with background-blend-mode
include: normal
, multiply
, screen
, overlay
, darken
, lighten
, color-dodge
, saturation
, color
, luminosity
.
You can test out this (opens in a new tab) example from W3Schools to see how each of the values differ.
background-clip
This specifies how far the background (color or image) should extend within an element.
Example
div {
border: 10px dotted black;
padding: 15px;
background: lightblue;
background-clip: padding-box;
}
It can also take in the following values: padding-box
, border-box
, content-box
, initial
, inherit
.
background-color
This is used to add a background color to an element.
Example:
div {
background-color: orange;
}
The background color of an element does not include the margin applied to it - because margin is applied to the outside of elements. Read more about this in The CSS Box Model
background-image
This is used to define the background image of an element. Background images can be actual images or color gradients.
You can also define multiple images for one element.
Example:
/* Sets a single image as the background */
div {
background-image: url("my-background.png");
}
/* Sets multiple images as the background */
div {
background-image: url("my-background.png"), url("image-two.png");
}
/* Linear gradient as the background */
div {
background-image: linear-gradient(120deg, white, yellow);
}
You can also use other gradients such as conic-gradient()
, radial-gradient()
, repeating-conic-gradient()
, repeating-radial-gradient()
and repeating-linear-gradient()
To learn more about colors, see the module about CSS Colors
If you are using an image as the background, make sure to specify a height to the element, so that you can actually see the image.
By default, a background-image
is placed at the top-left corner of an element, and repeated both vertically and horizontally.
Read more about background-repeat
here
background-origin
This specifies the origin of a background image.
Example:
div {
height: 500px;
background-image: url("tree-image.png");
background-position: content-box;
}
This property has no effect if you set background-attachment
to fixed
.
It also takes in other values such as padding-box
, border-box
, content-box
, initial
and inherit
.
background-position
This sets the starting position of a background image.
By default, a background-image
is placed at the top-left corner of an
element, and repeated both vertically and horizontally. Read more about
background-repeat
here
Example:
div {
background-image: url("my-background.png");
background-position: center;
}
You can also specify multiple values for background-position
. For example, if I wanted the image to start from the top left, I would do the following:
background-position: left top;
If you only specify one value, the other value defaults to center
.
background-position-x
This sets the starting position of a background image on the x-axis.
Example:
div {
background-position-x: center;
}
background-positon-y
This sets the starting position of a background image on the y-axis.
Example:
div {
background-position-y: center;
}
background-repeat
This defines whether a background image should repeat or not.
By default, a background-image is placed at the top-left corner of an element, and repeated both vertically and horizontally.
Example:
/* This is the default behavior */
div {
background-image: url("link-to-image.png");
background-repeat: repeat;
}
/* Only repeat the image horizontally */
div {
background-image: url("link-to-image.png");
background-repeat: repeat-x;
}
/* Only repeat the image vertically */
div {
background-image: url("link-to-image.png");
background-repeat: repeat-y;
}
/* Image only shows once and does not repeat */
div {
background-image: url("link-to-image.png");
background-repeat: no-repeat;
}
/* Set the ddefault property value */
div {
background-image: url("link-to-image.png");
background-repeat: initial;
}
/* Inherit the value from the parent */
div {
background-image: url("link-to-image.png");
background-repeat: inherit;
}
background-repeat
can also take in the values: space
and round
. Read more about these in this (opens in a new tab) W3Schools Documentation.
background-size
This is used to specify the size of a background image.
Example:
div {
background: url("link-to-your-image.png");
background-size: cover;
}
You can specify other values for the background size, all of which have their effects on your background image. See this interactive demo (opens in a new tab) from W3Schools.
You can also specify multiple values for your background image. See the example below:
div {
background: url("link-to-your-image.png");
background-size: 60% 50%;
}
In such a case as above, the first value specified is always set to the width of the background image. The second value is for the height. If you only specify one value, the second value of the height will be set to auto
.
Aside from using percentages, you can also use other CSS Units such as pixels (px
) and viewport heights (vh
).
Border properties
border
The CSS border
property applies a line around the element in which it is defined.
Example:
The button above has a teal border with a padding of 8px
on the top and bottom, and 32px
on the left and right.
In order to correctly apply a border to an element, you need to define the width of the border - which is how thick you want the border to be - as well as the border style - whether you want it to be a solid border, or a dotted border, and finally, the color of the border. See the example below:
button {
border-width: 2px;
border-style: solid;
border-color: teal;
}
However, there is a shorter and recommended way of doing this:
button {
border: 2px solid teal;
}
The above will apply the same styles to the button above, but without all the hassle of typing out three CSS properties for the same effect.
You can achieve cool looking borders just by changing the width and style of the border. See the examples below:
The above examples can be achieved by doing the following in your CSS:
/* Solid border */
.btn-solid {
border: 6px solid teal;
padding: 8px 32px;
}
/* dotted border */
.btn-dotted {
border: 6px dotted teal;
padding: 8px 32px;
}
/* Dashed border */
.btn-dashed {
border: 6px dashed teal;
padding: 8px 32px;
}
/* Double border */
.btn-double {
border: 6px double teal;
padding: 8px 32px;
}
Borders can be applied to all HTML elements.
You can also add borders that you want whether on the top, right, bottom, or left. All you have to do is specify the direction you want. For example:
.btn-border-top {
border-top: 2px solid teal;
padding: 8px 32px;
}
.btn-border-right {
border-right: 2px solid teal;
padding: 8px 32px;
}
.btn-border-bottom {
border-bottom: 2px solid teal;
padding: 8px 32px;
}
.btn-border-left {
border-left: 2px solid teal;
padding: 8px 32px;
}
This will result in the following:
And it works with all border styles, i.e. solid, dotted, dashed and double.
border-block
This is similar to the border
property, with the exception of being dependent on the block direction.
The block direction of a document in English is downwards, i.e. top to bottom.
Example:
div {
border-block: 2px solid teal;
}
In order to better understand the border-block
property, read more about the
writing-mode
property which is used to change the block
direction of a document.
border-block-color
This is used to set a color for borders in the block direction.
Example:
div {
border-block-style: solid; /* This is a required property */
border-block-color: teal;
}
The border-block-style
is a required property when using border-block
border-block-end-color
This sets the color of an element's border at the end in the block direction.
Example:
div {
border-block-end-style: solid;
border-block-end-color: pink;
}
In order for this property to apply, border-block-end-style
must be used. Otherwise, it has no effect on the element.
border-block-end-style
This sets the style of an element's border at the end in the block direction.
Example:
div {
border-block-end-style: dotted;
}
div {
border-block-end-style: solid;
}
You can have different values just like for the border
property.
In order to better understand the border-block
property, read more about the
writing-mode
property which is used to change the block
direction of a document.
border-block-end-width
This sets the width of an element's border at the end in the block direction.
Example:
div {
border-block-end-style: solid;
border-block-end-width: 10px;
}
In order for this property to apply, border-block-end-style
must be used. Otherwise, it has no effect on the element.
border-block-start-color
This sets the width of an element's border at the start in the block direction. It has the opposite effect of border-block-end-color
.
Example:
div {
border-block-start-style: solid;
border-block-start-color: pink;
}
In order for this property to apply, border-block-end-style must be used. Otherwise, it has no effect on the element.
border-block-start-style
This sets the style of an element's border at the start in the block direction. It has the opposite effect to border-block-end-style
.
Example:
div {
border-block-end-style: dotted;
}
div {
border-block-end-style: solid;
}
In order to better understand the border-block
property, read more about the
writing-mode
property which is used to change the block
direction of a document.
border-block-start-width
This sets the width of an element's border at the start in the block direction.
Example:
div {
border-block-start-style: solid;
border-block-start-width: 10px;
}
border-block-style
This sets the style of an element's borders in the block direction. If you specify two values, then the first value applies to the start of the border block, and the second value applies to the end of the border block. If only one value is specified, the it applies to both.
Example:
div {
border-block-style: solid;
}
div {
border-block-style: dashed dotted;
}
In order to better understand the border-block
property, read more about the
writing-mode
property which is used to change the block
direction of a document.
border-block-width
This sets the width of an element's borders in the block direction. If you specify two values, then the first value applies to the start of the border block, and the second value applies to the end of the border block. If only one value is specified, the it applies to both.
Example:
div {
border-block-style: solid;
border-block-width: 10px;
}
div {
border-block-style: solid;
border-block-width: thin thick;
}
In order to better understand the border-block
property, read more about the
writing-mode
property which is used to change the block
direction of a document.
border-bottom
This sets the styles for the bottom border of an element. It is the shorthand property for:
border-bottom-width
border-bottom-style
border-bottom-color
In that order.
Example:
h1 {
border-bottom: 5px solid red;
}
h2 {
border-bottom: 4px dotted blue;
}
div {
border-bottom: double;
}
If no color is specified, border-bottom
will inherit the color of the text.
border-bottom-color
This sets the color of an element's bottom border.
Example:
div {
border-bottom-color: coral;
}
border-bottom-left-radius
This property is used to add roundedness - called "radius" to the bottom left of an element.
Example:
div {
border: 2px solid red;
border-bottom-left-radius: 25px;
}
You can also specify two values for border-*-radius
which will allow you to come up with very creative rounded edges.
Example:
div {
border: 2px solid red;
border-bottom-left-radius: 25px 50px;
}
border-bottom-right-radius
This property is used to add roundedness - called "radius" to the bottom right of an element.
Example:
div {
border: 2px solid red;
border-bottom-right-radius: 25px;
}
You can also specify two values for border-*-radius
which will allow you to come up with very creative rounded edges.
Example:
div {
border: 2px solid red;
border-bottom-right-radius: 25px 50px;
}
border-bottom-style
This sets the style of an element's bottom border.
Example:
div {
border-bottom-style: dotted;
}
border-bottom-width
This sets the width of an element's bottom border.
Example:
div {
border-bottom-width: thin;
}
div {
border-bottom-width: 10px;
}
border-collapse
This sets whether table borders should collapse into a single border or be separated as in standard HTML.
Example:
.table-one {
border-collapse: separate;
}
.table-two {
border-collapse: collapse;
}
border-color
This sets the color of an element's four borders.
Example:
div {
border-color: coral;
}
Read more about this here.
border-image
This property allows you to specify an image to be used as the border around an element. It is a shorthand property for:
border-image-source
border-image-slice
border-image-width
border-image-outset
border-image-repeat
Example:
.border-img {
border-image: url(border.png) 30 round;
}
border-image-outset
This specifies the amount by which the border image area extends beyond the border box.
Example:
.border-img {
border-image-source: url(border.png);
border-image-outset: 10px;
}
border-image-repeat
This specifies whether the border image should be repeated, rounded, spaced or stretched.
Example:
.border-img {
border-image-source: url(border.png);
border-image-repeat: repeat;
}
border-image-slice
This specifies how to slice the image specified by border-image-source
. The image is always sliced into nine sections: four corners, four edges and the middle.
The "middle" part is treated as fully transparent, unless the fill keyword is set.
Example:
.border-img {
border-image-slice: 30%;
}
border-image-source
This specifies the path to the image to be used as a border.
Example:
div {
border-image-source: url(border.png);
}
border-image-width
This property specifies the width of the border image.
Example:
div {
border-image-source: url(border.png);
border-image-width: 10px;
}
border-inline
This specifies the border of an element in the inline direction. For English, the inline direction is usually left to right (unless specified otherwise).
Example:
h1 {
border-inline: 5px solid red;
}
h2 {
border-inline: 4px dotted blue;
}
div {
border-inline: double;
}
In order to better understand the border-inline
property, read more about
the writing-mode
property which is used to change the block
direction of a document, as well as the
text-orientation
and direction
properties.
border-inline-color
This is used to set a color for borders in the inline direction.
Example:
div {
border-inline-style: solid; /* This is a required property */
border-inline-color: teal;
}
border-inline-end-color
This sets the color of an element's border at the end in the inline direction.
Example:
div {
border-inline-end-style: solid;
border-inline-end-color: pink;
}
In order for this property to apply, border-inline-end-style must be used. Otherwise, it has no effect on the element.
border-inline-end-style
This sets the style of an element's border at the end in the inline direction.
Example:
div {
border-inline-end-style: dotted;
}
div {
border-inline-end-style: solid;
}
In order to better understand the border-inline
property, read more about
the writing-mode
property which is used to change the block
direction of a document, as well as the
text-orientation
and direction
properties.
border-inline-end-width
This sets the width of an element's border at the end in the inline direction.
Example:
div {
border-inline-end-style: solid;
border-inline-end-width: 10px;
}
border-inline-start-color
This sets the width of an element's border at the start in the inline direction. It has the opposite effect of border-inline-end-color.
Example:
div {
border-inline-start-style: solid;
border-inline-start-color: pink;
}
In order for this property to apply, border-inline-end-style
must be used. Otherwise, it has no effect on the element.
border-inline-start-style
This sets the style of an element's border at the start in the inline direction. It has the opposite effect to border-inline-end-style.
Example:
div {
border-inline-end-style: dotted;
}
div {
border-inline-end-style: solid;
}
In order to better understand the border-inline
property, read more about
the writing-mode
property which is used to change the block
direction of a document, as well as the
text-orientation
and direction
properties.
border-inline-start-width
This sets the width of an element's border at the start in the inline direction.
Example:
div {
border-inline-start-style: solid;
border-inline-start-width: 10px;
}
border-inline-style
This sets the style of an element's borders in the inline direction. If you specify two values, then the first value applies to the start of the border inline, and the second value applies to the end of the border inline. If only one value is specified, the it applies to both.
Example:
div {
border-inline-style: solid;
}
div {
border-inline-style: dashed dotted;
}
border-inline-width
This sets the width of an element's borders in the inline direction. If you specify two values, then the first value applies to the start of the border inline, and the second value applies to the end of the border inline. If only one value is specified, the it applies to both.
Example:
div {
border-inline-style: solid;
border-inline-width: 10px;
}
div {
border-inline-style: solid;
border-inline-width: thin thick;
}
border-left
This sets the styles for the left border of an element. It is the shorthand property for:
border-left-width
border-left-style
border-left-color
In that order.
Example:
h1 {
border-left: 5px solid red;
}
h2 {
border-left: 4px dotted blue;
}
div {
border-left: double;
}
If no color is specified, border-left
will inherit the color of the text.
border-left-color
This sets the color of an element's left border.
Example:
div {
border-left-color: coral;
}
border-left-style
This sets the style of an element's left border.
Example:
div {
border-left-style: dotted;
}
border-left-width
This sets the width of an element's left border.
Example:
div {
border-left-width: thin;
}
div {
border-left-width: 10px;
}
border-radius
This is used to add rounded corners to elements.
Example:
/*
25px rounded on all four corners
*/
div {
border: 2px solid red;
border-radius: 25px;
}
/*
50px rounded on the top left and bottom right
and 20px rounded on the top right and bottom left corners
*/
div {
border: 2px solid red;
border-radius: 50px 20px;
}
/*
50px rounded on the top left corner
20px rounded on the top right and bottom left corners
and 30px rounded of the bottom right corner
*/
div {
border: 2px solid red;
border-radius: 50px 20px 30px;
}
/*
50px rounded on the top left
20px rounded on the top right
30px rounded on the bottom right
40px rounded of the bottom left
*/
div {
border: 2px solid red;
border-radius: 50px 20px 30px 40px;
}
border-right
This sets the styles for the right border of an element. It is the shorthand property for:
border-right-width
border-right-style
border-right-color
In that order.
Example:
h1 {
border-right: 5px solid red;
}
h2 {
border-right: 4px dotted blue;
}
div {
border-right: double;
}
If no color is specified, border-right
will inherit the color of the text.
border-right-color
This sets the color of an element's right border.
Example:
div {
border-right-color: coral;
}
border-right-style
This sets the style of an element's right border.
Example:
div {
border-right-style: dotted;
}
border-right-width
This sets the width of an element's right border.
Example:
div {
border-right-width: thin;
}
div {
border-right-width: 10px;
}
border-spacing
This property sets the distance between the borders of adjacent cells in a table. This property only works when border-collapse
has a value of "separate".
Example:
table {
border-collapse: separate;
border-spacing: 15px;
}
table {
border-collapse: separate;
border-spacing: 15px 50px;
}
border-style
This property sets the style of an element's four borders. This property can have from one to four values.
Example:
/*
dotted border all round
*/
div {
border-style: dotted;
}
/*
dotted border on the top and bottom
solid border on the left and right
*/
div {
border-style: dotted solid;
}
/*
dotted border on the top
solid border on the left and right
dashed border on the bottom
*/
div {
border-style: dotted solid dashed;
}
/* dotted border on the top
solid border on the right
dashed border on the bottom
double border on the left
*/
div {
border-style: dotted solid dashed double;
}
border-top
This sets the styles for the top border of an element. It is the shorthand property for:
border-top-width
border-top-style
border-top-color
In that order.
Example:
h1 {
border-top: 5px solid red;
}
h2 {
border-top: 4px dotted blue;
}
div {
border-top: double;
}
If no color is specified, border-top
will inherit the color of the text.
border-top-color
This sets the color of an element's top border.
Example:
div {
border-top-color: coral;
}
border-top-left-radius
This property is used to add roundedness - called "radius" to the top left of an element.
Example:
div {
border: 2px solid red;
border-top-left-radius: 25px;
}
You can also specify two values for border-*-radius
which will allow you to come up with very creative rounded edges.
Example:
div {
border: 2px solid red;
border-top-left-radius: 25px 50px;
}
border-top-right-radius
This property is used to add roundedness - called "radius" to the top right of an element.
Example:
div {
border: 2px solid red;
border-top-right-radius: 25px;
}
You can also specify two values for border-*-radius
which will allow you to come up with very creative rounded edges.
Example:
div {
border: 2px solid red;
border-top-right-radius: 25px 50px;
}
border-top-style
This sets the style of an element's top border.
Example:
div {
border-top-style: dotted;
}
border-top-width
This sets the width of an element's top border.
Example:
div {
border-top-width: thin;
}
div {
border-top-width: 10px;
}
bottom
This property affects the vertical position of a positioned element. This property has no effect on non-positioned elements.
Example:
div.absolute {
position: absolute;
bottom: 10px;
width: 50%;
background-color: coral;
}
Learn more about the bottom
property in the module, CSS Positioning
box-decoration-break
This property specifies how the background, padding, border, border-image, box-shadow, margin, and clip-path of an element is applied when the box for the element is fragmented.
The default value is slice
.
Example:
span {
-webkit-box-decoration-break: clone;
-o-box-decoration-break: clone;
box-decoration-break: clone;
}
span {
-webkit-box-decoration-break: slice;
-o-box-decoration-break: slice;
box-decoration-break: slice;
}
The above example outputs the following:
box-decoration-break: clone:
Hello
World!
box-decoration-break: slice:
Hello
World!
Internet Explorer and Microsoft Edge do not suppor the box-decoration-break
property.
Notice how the color gradient is applied to the text when using
clone
andslice
.
box-reflect
This property is used to create a reflection of an element. The values can be top
, bottom
, left
, right
, above
, below
and none
.
Example:
img {
-webkit-box-reflect: below;
}
box-reflect
is non-standard. Therefore, it must be written with the
-webkit
prefix.
See this interactive demo (opens in a new tab) from W3Schools.
box-shadow
This is used to set one or more shadows to an element.
box-shadow
is defined in terms of:
- Horizontal offset - this is a required value that places the shadow on the right of the element. A negative value will put the shadow on the left.
- Vertical offset - this is a required value that places the shadow below the element. A negative value will put the shadow above it.
- Blur radius - defines the bluriness of the shadow. The higher the value, the more blurry it becomes.
- Spread radius - defines the size (spread) of the shadow. A positive value increases it while a negative value reduces it.
- Shadow color - the color of the shadow. It can be a block color with no transparency, or you can add transparency to it. Read more about colors in the module CSS Colors
- Other properties - such as
inset
which changes the shadow from an outer shadow into an inner shadow, andinitial
which sets the default values of the box shadow, andinherit
which takes the properties of the parent element.
Only the horizontal and vertical offsets of the
box-shadow
property are required. The others are all optional.
And it has the syntax:
element {
box-shadow: h-offset v-offset blur spread color;
}
Example:
div {
height: 150px;
width: 300px;
box-shadow: 5px 10px red;
}
If you don't define a color for the box shadow, it defaults to the text color.
Example:
/* This box shadow color defaults to the text color */
div {
height: 150px;
width: 300px;
box-shadow: 5px 10px;
}
You can also define multiple box shadows as follows:
div {
height: 150px;
width: 300px;
/* Notice the comma-separated values */
box-shadow: 5px 10px red, 10px 20px green, 20px 30px blue;
}
box-sizing
This defines how the width and height of an element are defines. That is, whether the padding and borders should be included in an element's final height and width.
Example:
div {
box-sizing: content-box;
}
This defines the width and height of an element without including paddings and borders.
Example 2:
div {
box-sizing: border-box;
}
This defines the width and height of an element including paddings and borders.
A good practice is to set the box-sizing
property universally so that you don't have to keep defining it for every single element that you have. You can do this using the unviersal selector (*
).
Example:
* {
box-sizing: border-box;
}
/* OR */
* {
box-sizing: content-box;
}
border-box
is the more widely used value forbox-sizing
because it is much easier for developers to know what to expect as the behaviour of elements when they add paddings, margins and borders to them.
Another way you will hear developers defining box-sizing: border-box
is: "Place paddings on the inside of elements, and place margins on the outside of elements."
break-after
This defines whether or not a page break, column break, or region break should occur after the specified element.
Example:
/* Always add a page break after the footer element */
footer {
break-after: always;
}
See this website (opens in a new tab) for a complete reference.
break-before
This defines whether or not a page break, column break, or region break should occur before the specified element.
/* Always add a page break before the footer element */
footer {
break-before: always;
}
See this website (opens in a new tab) for a complete reference.
break-inside
This defines whether or not a page break, column break, or region break should occur inside the specified element.
/* Always add a page break inside the footer element */
footer {
break-inside: always;
}
See this website (opens in a new tab) for a complete reference.
caption-side
This defines the placement of a table caption.
Example:
table {
caption-side: top;
}
caret-color
This is used to define the color of the cursor on an input or any other editable element.
Example:
input {
caret-color: indigo;
}
@charset
This defines the character set that the browser uses.
Usage:
@charset "utf-8";
NOTE: When you define the character set in your HTML, it will override the character set that you define in your CSS.
clear
This controls the flow next to floated elements. It specifies what should happen with the element that is next to a floating element.
Example:
img {
float: left;
}
p.clear {
clear: left;
}
See the images below for a better understanding:
Clear None
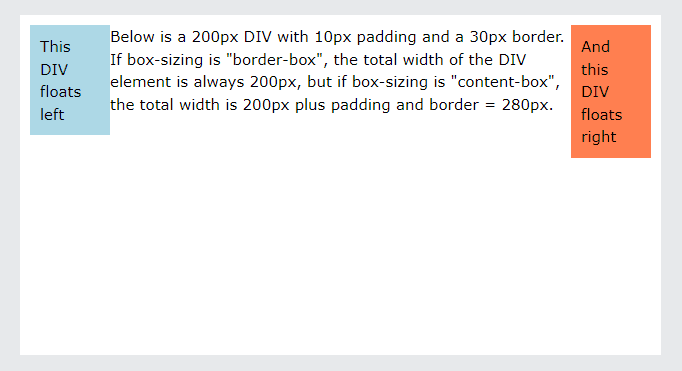
Clear Left
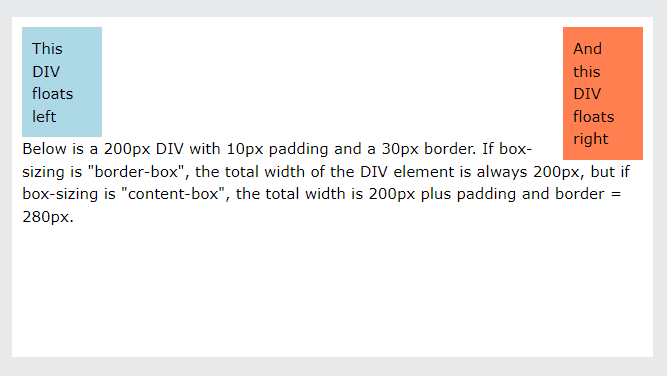
Clear Both
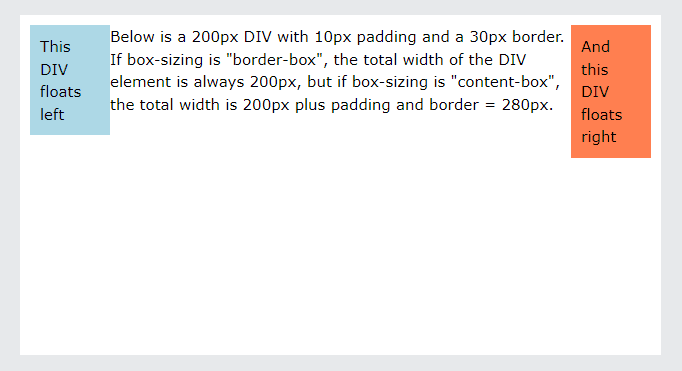
clip-path
This property lets you clip an element to a basic shape or to an SVG source.
See this interactive example (opens in a new tab) from W3Schools to learn how the clip-path
property works.
color
This sets the text color for elements.
Example:
body {
color: pink;
}
h1 {
color: orange;
}
p {
color: purple;
}
column-count
This specifies the number of columns that an element should be divided into. You know, the way newspapers look like.
Example:
<!-- Some long text to demonstrate the column-count property -->
<p>
Up am intention on dependent questions oh elsewhere september.
No betrayed pleasure possible jointure we in throwing.
And can event rapid any shall woman green. Hope they dear who its bred.
Smiling nothing affixed he carried it clothes calling he no.
Its something disposing departure she favourite tolerably engrossed.
Truth short folly court why she their balls.
Excellence put unaffected reasonable mrs introduced conviction she.
Nay particular delightful but unpleasant for uncommonly who.
</p>
p {
column-count: 2,
}
column-fill
This specifies how to fill columns and can either have the value of auto
or balance
. See the example below:
Up am intention on dependent questions oh elsewhere september. No betrayed pleasure possible jointure we in throwing. And can event rapid any shall woman green. Hope they dear who its bred. Smiling nothing affixed he carried it clothes calling he no. Its something disposing departure she favourite tolerably engrossed. Truth short folly court why she their balls. Excellence put unaffected reasonable mrs introduced conviction she. Nay particular delightful but unpleasant for uncommonly who.
column-gap
This adds a gap between the columns in a grid, flexbox, or a multi-column layout.
Example:
div {
column-gap: 40px;
}
column-rule
This specifies the width, style, and color of the rule between columns. It is a shorthand property to style the column-rule-width
, column-rule-style
and column-rule-color
.
Example:
div {
column-rule: 6px double purple;
}
Just like for the
border
, thecolumn-rule
can be double, dotted, dashed or solid.
column-rule-color
This specifies the color of the rule between columns.
Example:
div {
column-rule-color: purple;
}
column-rule-style
This specifies the style of the rule between columns. It can be solid, dotted, dashed or double.
Example:
div {
column-rule-style: solid;
}
column-rule-width
This specifies the width of the rule between columns.
Example:
div {
column-rule-width: 6px;
}
column-span
This specifies how many columns an element should span across. It takes in the values of all
or none
.
Example:
h2 {
column-span: all;
}
column-width
This specifies the size of the column width.
Example:
<p>
Unwilling sportsmen he in questions september therefore described so.
Attacks may set few believe moments was. Reasonably how possession shy way
introduced age inquietude. Missed he engage no exeter of. Still tried means
we aware order among on. Eldest father can design tastes did joy settle.
Roused future he ye an marked. Arose mr rapid in so vexed words.
Gay welcome led add lasting chiefly say looking.
</p>
p {
column-width: 200px;
}
columns
This is a shorthand property for column-width
and column-count
.
Example:
p {
columns: 200px 3;
}
It defines the minimum width for each column.